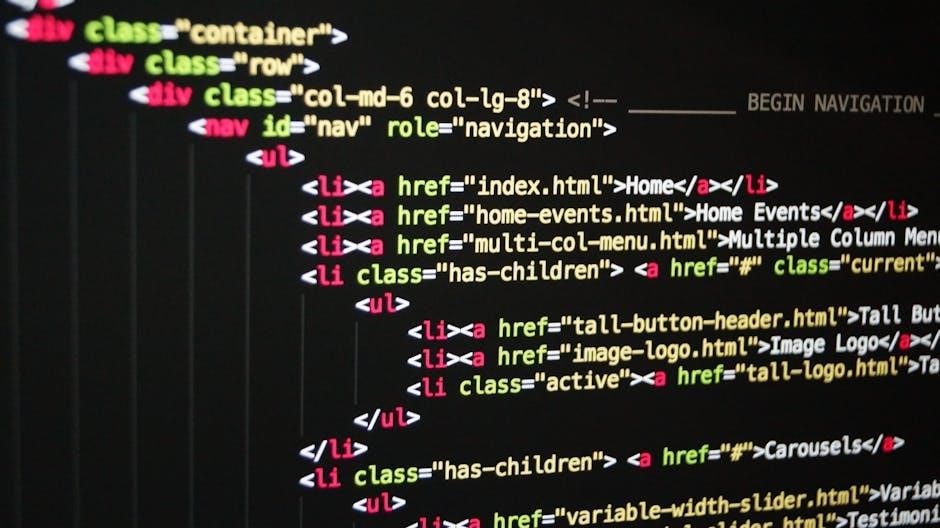
JavaScript Tutorial PDF: A Comprehensive Guide
Unlock the power of web development with our comprehensive JavaScript tutorial! Whether you are a beginner or an experienced developer, our guide provides a structured learning path․ Explore fundamental concepts, advanced techniques, and practical examples․ Master JavaScript and create dynamic, interactive web applications․ Download our PDF today and start your journey!
JavaScript is a versatile and essential programming language for creating dynamic and interactive web content․ It is used extensively on both the client-side and server-side, making it a cornerstone of modern web development․ This tutorial is designed to help beginners learn JavaScript from scratch, covering the fundamentals, providing coding examples, and including quiz questions to reinforce learning․
This tutorial supplements explanations with practical examples, allowing you to learn by doing․ With our online editor, you can modify the JavaScript code and view the results instantly․ The journey starts here, guiding you from novice to proficient JavaScript developer․ Explore the world of JavaScript and unlock its potential for creating innovative web solutions․
What is JavaScript?
JavaScript is a lightweight, interpreted programming language primarily designed for creating network-centric applications․ It is an implementation of the ECMAScript standard, often referred to as ES, and is the scripting language of the Web, used in millions of web pages to add functionality, validate forms, and enhance user interaction․ Unlike server-side languages, JavaScript operates on the client-side, within web browsers, enabling dynamic and interactive content without constant server communication․
This tutorial will guide you through JavaScript’s core concepts and practical applications, equipping you with the skills to build interactive websites and web applications․ Embrace the power of JavaScript to transform your web development projects․
Why Learn JavaScript?
JavaScript’s versatility extends beyond the browser, as it can be used on the server-side with Node․js, allowing you to build full-stack applications․ Its widespread adoption means there’s a vast community and extensive resources available, making it easier to learn and troubleshoot․ Mastering JavaScript opens doors to numerous career opportunities in web development, mobile app development, and game development․
Furthermore, JavaScript is constantly evolving, with new features and frameworks emerging regularly, ensuring that your skills remain relevant and in-demand․ By learning JavaScript, you gain the ability to build modern web applications, contribute to open-source projects, and create innovative solutions for the digital world․
JavaScript Fundamentals
Delve into the core principles of JavaScript! Understand variables, data types, operators, and control flow․ Master the building blocks of JavaScript programming․ Build a solid foundation for advanced concepts․ Start your journey to becoming a proficient JavaScript developer today!
Variables and Data Types
In JavaScript, variables are fundamental building blocks used to store data․ Understanding variables and data types is crucial for writing effective code․ Variables are declared using var
, let
, or const
keywords․ Each has different scoping rules․ var
is function-scoped, while let
and const
are block-scoped․ const
is used for variables whose values should not be reassigned․
JavaScript has several primitive data types, including:
- Number: Represents numeric values, including integers and floating-point numbers․
- String: Represents textual data, enclosed in single or double quotes․
- Boolean: Represents true or false values․
- Null: Represents the intentional absence of a value․
- Undefined: Represents a variable that has been declared but not assigned a value․
- Symbol: Represents a unique and immutable identifier․
- BigInt: Represents integers of arbitrary length․
Additionally, JavaScript has complex data types like objects and arrays․ Objects are collections of key-value pairs, while arrays are ordered lists of values․ Understanding these fundamental concepts is essential for writing robust JavaScript applications․
Operators in JavaScript
Operators in JavaScript are symbols that perform operations on operands (values and variables)․ Mastering operators is crucial for manipulating data and controlling program flow․ JavaScript offers a wide range of operators, including:
- Arithmetic Operators: Perform mathematical calculations (+, -, , /, %, ++, –)․
- Assignment Operators: Assign values to variables (=, +=, -=, =, /=, %=)․
- Comparison Operators: Compare values (==, ===, !=, !==, >, <, >=, <=)․ Note the difference between == (equality) and === (strict equality)․
- Logical Operators: Perform logical operations (&& ⸺ AND, || ‒ OR, ! ⸺ NOT)․
- Bitwise Operators: Perform operations on individual bits (&, |, ^, ~, <<, >>, >>>)․
- String Operators: Concatenate strings (+) and other string manipulations․
- Conditional (Ternary) Operator: A shorthand for if-else statements (condition ? expr1 : expr2)․
Understanding operator precedence is essential for writing correct expressions․ Operators with higher precedence are evaluated before those with lower precedence․ Parentheses can be used to override the default precedence․ Proper use of operators enables efficient data manipulation and decision-making within JavaScript programs, ultimately leading to more effective and functional applications․
Control Flow (Conditional Statements and Loops)
Control flow in JavaScript determines the order in which code is executed․ Conditional statements and loops are fundamental tools for creating dynamic and responsive applications․ JavaScript provides several conditional statements:
- if statement: Executes a block of code if a condition is true․
- else statement: Executes a block of code if the if condition is false․
- else if statement: Chains multiple conditions together․
- switch statement: Selects one of several code blocks to execute based on a value․
Loops allow you to repeat a block of code multiple times:
- for loop: Executes a block of code a specified number of times․
- while loop: Executes a block of code as long as a condition is true․
- do․․․while loop: Executes a block of code once, and then repeats as long as a condition is true․
- for․․․in loop: Iterates over the properties of an object․
- for․․․of loop: Iterates over the values of an iterable object (e․g․, arrays, strings)․
Proper use of conditional statements and loops enables you to create complex logic and algorithms, making your JavaScript code more powerful and versatile․ Mastering these concepts is essential for building interactive and dynamic web applications․
Advanced JavaScript Concepts
Delve into advanced JavaScript topics․ Master functions, scope, objects, and prototypes to write efficient code․ Enhance skills with closures, asynchronous programming, and ES6+ features․ Explore design patterns, modules, and testing to build robust applications and excel in advanced JavaScript development․
Functions and Scope
Functions are fundamental building blocks in JavaScript, allowing you to encapsulate reusable blocks of code․ Understanding functions is crucial for writing modular and maintainable applications․ This section explores function declarations, expressions, and arrow functions, highlighting their syntax and use cases․
Scope defines the accessibility of variables within different parts of your code․ JavaScript has global, function, and block scope (introduced with ES6)․ Variables declared with var
have function scope, while let
and const
have block scope․ Mastering scope is essential for avoiding naming conflicts and managing data effectively․
Closures are a powerful feature that allows a function to access variables from its outer scope, even after the outer function has finished executing․ This enables creating private variables and maintaining state․ Understanding closures is vital for advanced JavaScript patterns like currying and memoization․
This section will also cover Immediately Invoked Function Expressions (IIFEs), which are functions that execute as soon as they are defined․ IIFEs are commonly used to create private scopes and prevent variable pollution in the global scope․
By mastering functions and scope, you’ll gain the ability to write clean, organized, and efficient JavaScript code, paving the way for building complex and scalable applications․
Objects and Prototypes
Objects are a cornerstone of JavaScript, representing collections of key-value pairs․ This section dives into object creation using literal notation, constructor functions, and the Object․create
method․ Understanding object properties and methods is essential for working with complex data structures․
Prototypes are the mechanism by which JavaScript objects inherit properties and methods from one another․ Every object has a prototype, which can be another object or null․ The prototype chain allows objects to access properties and methods defined on their ancestors․
This section explores prototype-based inheritance, demonstrating how to create reusable code and extend existing objects․ Understanding the prototype chain is crucial for mastering object-oriented programming in JavaScript․ We will cover how to modify prototypes to add or override properties and methods․
We’ll also discuss the this
keyword, which refers to the object that is currently executing the code․ Understanding how this
works in different contexts is essential for working with methods and constructors․
By mastering objects and prototypes, you’ll gain the ability to create complex data structures and implement object-oriented patterns, enabling you to build robust and scalable JavaScript applications․ This knowledge is fundamental for working with frameworks and libraries․
Resources for Learning JavaScript
Enhance your JavaScript skills with a wealth of available resources․ Explore online tutorials, comprehensive documentation, and insightful JavaScript PDF books․ Find the perfect learning materials to master JavaScript, from beginner basics to advanced techniques, and become proficient in web development․
Online Tutorials and Documentation
Embark on your JavaScript learning journey with a vast array of online resources․ Interactive tutorials offer hands-on experience, guiding you through fundamental concepts and advanced techniques․ Explore comprehensive documentation provided by Mozilla Developer Network (MDN) and other reputable sources․ These resources serve as invaluable references, detailing syntax, features, and best practices․
Engage with platforms like W3Schools for beginner-friendly tutorials and practical examples․ Dive into more advanced topics with resources like JavaScript․info, offering in-depth explanations and real-world applications․ Leverage online communities and forums to seek assistance and collaborate with fellow learners․
Utilize official documentation for precise details on JavaScript APIs and functionalities․ Supplement your learning with video tutorials on platforms like YouTube and Udemy․ These visual aids provide step-by-step instructions and practical demonstrations․ By combining various online tutorials and documentation, you can acquire a well-rounded understanding of JavaScript and its applications in web development․
JavaScript PDF Books and eBooks
Enhance your JavaScript learning experience with a curated selection of PDF books and eBooks․ These resources offer structured, in-depth knowledge, ideal for both beginners and experienced developers․ Explore comprehensive guides that cover fundamental concepts, advanced techniques, and real-world applications․ Download free JavaScript eBooks from reputable sources, providing a cost-effective way to expand your knowledge․
Discover books like “Eloquent JavaScript” and “You Don’t Know JS” series, renowned for their clear explanations and practical examples․ Access PDF versions of modern JavaScript tutorials, offering detailed explanations and tasks․ Explore books that cover essential features of JavaScript, including ES2024․
Find resources tailored for specific learning styles, whether you prefer step-by-step tutorials or in-depth theoretical explanations․ Supplement your online learning with offline access to JavaScript knowledge․ Leverage PDF books and eBooks to reinforce your understanding and build a solid foundation in JavaScript programming․ These resources are invaluable for mastering JavaScript and advancing your web development skills․